반응형
이전 글에서 조금 발전해서, 프로세스 목록을 listbox로 출력하고 그중에 선택하는 방법
1. ListBox 사용
1) 기본 사용법
요 글 참고해서 연습함.
//xaml
<ListBox Name="lbWindowsList" HorizontalAlignment="Left" Height="326" Margin="105,0,0,0" VerticalAlignment="Center" Width="209">
<ListBox.ItemTemplate>
<DataTemplate>
<TextBlock Text="{Binding Title}"/>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
//code side
private void Button_Click(object sender, RoutedEventArgs e)
{
List<WindowList> items = new List<WindowList>();
items.Add(new WindowList() { Title = "Title 1" });
items.Add(new WindowList() { Title = "Title 2" });
items.Add(new WindowList() { Title = "Title 3" });
lbWindowsList.ItemsSource = items;
}
public class WindowList
{
public string Title { get; set; }
}
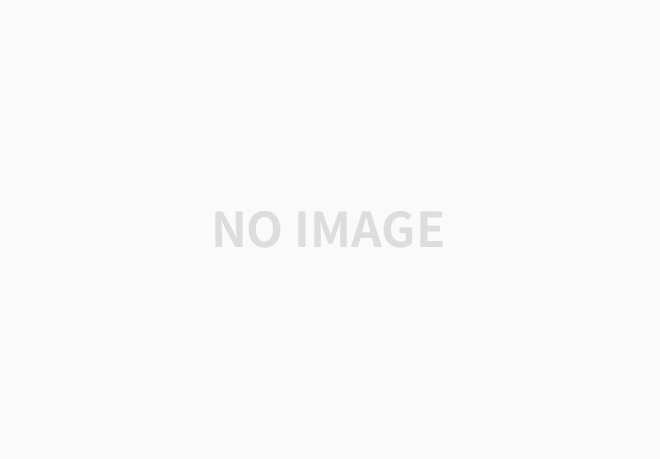
2) 프로세스들을 ListBox에 넣기
이전 글에서 프로세스 목록을 뽑는건 해봤고, 이걸 listbox의 item에 추가.
//xaml
<ListBox Name="lbWindowsList" HorizontalAlignment="Left" Height="326" Margin="105,0,0,0" VerticalAlignment="Center" Width="209">
<ListBox.ItemTemplate>
<DataTemplate>
<TextBlock Text="{Binding Title}"/>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
//code side
private void Button_Click(object sender, RoutedEventArgs e)
{
List<WindowList> items = new List<WindowList>();
//items.Add(new WindowList() { Title = "Title 1" });
//items.Add(new WindowList() { Title = "Title 2" });
//items.Add(new WindowList() { Title = "Title 3" });
lbWindowsList.ItemsSource = items;
ArrayList list = GetWindows();
Debug.WriteLine(list.Count);
StringBuilder winText = new StringBuilder();
int winTitleLength = 0;
int count = 0;
int length = 0;
foreach (IntPtr _winHandle in list)
{
try
{
winTitleLength = GetWindowTextLength(_winHandle); //윈도 타이틀의 길이 가져옴.
winText.Capacity = winTitleLength; //타이틀 길이에 맞춰 stringbulder 크기 조절
count++;
GetWindowText(_winHandle, winText, winTitleLength);
//Debug.WriteLine(count + ": " + _winHandle.ToString());
}
finally
{
string _process = count + " " + _winHandle + ": " + winText;
items.Add(new WindowList() { Title = _process });
Debug.WriteLine(_process);
winText.Clear();
}
}
}
public class WindowList
{
public string Title { get; set; }
}
//user32.dll import 함수들
public delegate bool EnumedWindow(IntPtr handleWindow, ArrayList handles);
[DllImport("user32.dll", CharSet = CharSet.Auto, SetLastError = true)]
[return: MarshalAs(UnmanagedType.Bool)]
public static extern bool EnumWindows(EnumedWindow lpEnumFunc, ArrayList lParam);
[DllImport("user32.dll", CharSet = CharSet.Auto, SetLastError = true)]
static extern int GetWindowText(IntPtr hWnd, StringBuilder lpString, int nMaxCount);
[DllImport("user32.dll", EntryPoint = "GetWindowTextLength", SetLastError = true)]
internal static extern int GetWindowTextLength(IntPtr hwnd);
public static ArrayList GetWindows()
{
ArrayList windowHandles = new ArrayList();
EnumedWindow callBackPtr = GetWindowHandle;
EnumWindows(callBackPtr, windowHandles);
return windowHandles;
}
private static bool GetWindowHandle(IntPtr windowHandle, ArrayList windowHandles)
{
windowHandles.Add(windowHandle);
return true;
}
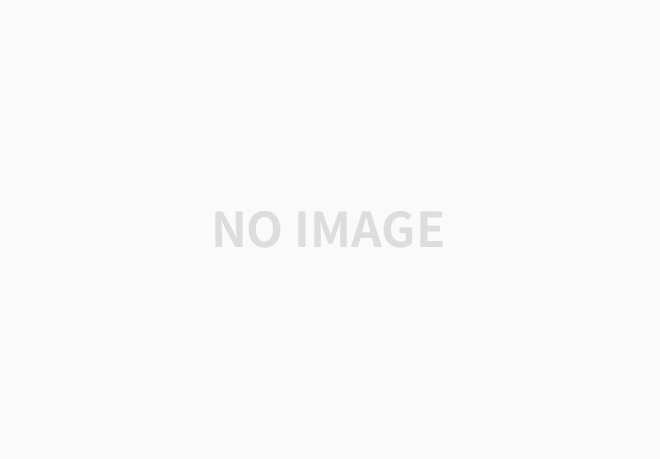
3) 특정 제목의 창 골라내기
원하는 제목의 창을 골라내는(filter) 기능 추가...
private void btn_FilterProcess(object sender, RoutedEventArgs e)
{
Debug.WriteLine("filter Test");
//listbox의 전체 아이템들을 불러옴
var processList = lbWindowsList.ItemsSource;
//새로운 아이템들이 들어갈 변수 선언
List<WindowList> processList_filtered = new List<WindowList>();
foreach (WindowList process in processList)
{
if (process.Title.Contains("메모"))
{
processList_filtered.Add(process);
Debug.WriteLine(process.Title);
}
}
//새로운 아이템 리스트로 교체
lbWindowsList.ItemsSource = processList_filtered;
}
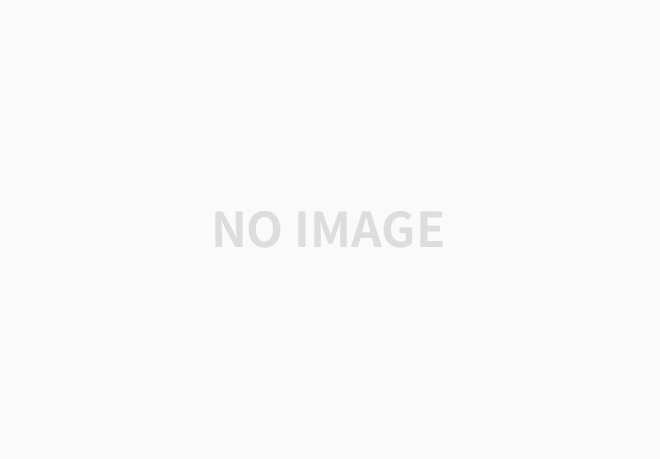
4) 선택한 프로세스 정보 출력
private void btn_SelectProcess(object sender, RoutedEventArgs e)
{
var selectedProcess = (WindowList)lbWindowsList.SelectedItems[0];
string _output = String.Format("Index: {0}, Handle: {1}, Title: {2}", selectedProcess.Index, selectedProcess.Handle, selectedProcess.Title);
Debug.WriteLine(_output);
}
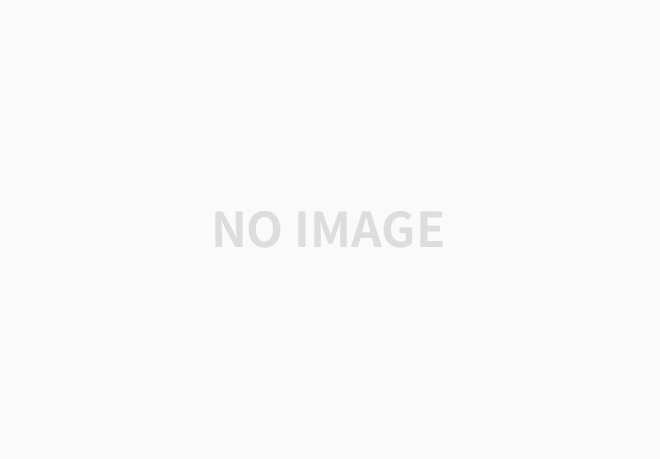
728x90
반응형
'C#' 카테고리의 다른 글
[C#] 이미지파일 읽기 + 출력 (Bitmapimage, ImageDrawing) (0) | 2021.01.03 |
---|---|
[C#] Thread - 반복실행, Timer (0) | 2020.11.26 |
[OpenCV] OpenCvSharp4 - 캡쳐 화면 클릭해서 좌표 얻기 (0) | 2020.11.05 |
[C#] Thread - thread 지정 + 상태확인 (0) | 2020.11.01 |
[C#] 화면캡쳐 + 출력 (OpenCvSharp4) (0) | 2020.10.22 |
최근댓글